|
1 | 1 | # ts-guideline
|
2 | 2 |
|
| 3 | + <font size="4"> |
| 4 | + |
3 | 5 | Minimalistic configuration for TS to only extend JS with types. No TS-scpecific features, no bundling. Readable maintainable code after compilation.
|
4 | 6 |
|
5 |
| - - **commonjs** folder for CommonJS Modules ts-configuration compatible with ECMAScript import |
| 7 | +- **commonjs** folder for CommonJS Modules ts-configuration compatible with ECMAScript import |
| 8 | + |
| 9 | +- **ecmascript** folder for ECMAScript Modules ts-configuration |
| 10 | + |
| 11 | +- **js-dts** folder for JS + DTS configuration |
| 12 | + |
| 13 | +- **js-doc** folder for JS Doc + TypeScript configuration |
| 14 | + |
| 15 | + </font> |
| 16 | + |
| 17 | +# Reccomendations |
| 18 | + |
| 19 | +<font size="4"> |
| 20 | + |
| 21 | +1. **Avoid** using **type aliases** - example from mongoose types |
| 22 | + |
| 23 | + ```ts |
| 24 | + export type ApplyBasicQueryCasting<T> = T | T[] | (T extends (infer U)[] ? U : any) | any; |
| 25 | + ``` |
| 26 | + |
| 27 | + this is unreadable and overcomplicated |
| 28 | + |
| 29 | + **Aliases are** good **only** for **simple** types |
| 30 | + |
| 31 | + ```ts |
| 32 | + type Fruit = 'banana' | 'orange' | 'pineapple' | 'watermelon'; |
| 33 | +
|
| 34 | + type Debt = { amount: number; dueTo: Date }; |
| 35 | + type AccountDebt = { accountId: number; debt: Debt | null }; |
| 36 | + ``` |
| 37 | + |
| 38 | +2. **Never use complicated generic types** - example from mongoose types |
| 39 | + ```ts |
| 40 | + type QueryWithHelpers<ResultType, DocType, THelpers = {}, RawDocType = DocType> = Query< |
| 41 | + ResultType, |
| 42 | + DocType, |
| 43 | + THelpers, |
| 44 | + RawDocType |
| 45 | + > & |
| 46 | + THelpers; |
| 47 | + ``` |
| 48 | + basically is is looks worse then |
| 49 | + ```ts |
| 50 | + type QueryWithHelpers<any> = Query<any> & any; |
| 51 | + ``` |
| 52 | +3. Not everything is neccessary to cover with types. |
| 53 | + |
| 54 | + It requires to add useless interfaces for type like this. Better just not use this. |
| 55 | + |
| 56 | + ```ts |
| 57 | + // with typing |
| 58 | + interface IDynamicGeneratedClass {} |
| 59 | +
|
| 60 | + const classGenerator = (): IDynamicGeneratedClass => |
| 61 | + class DynamicGeneratedClass implements IDynamicGeneratedClass { |
| 62 | + args: number[]; |
| 63 | + constructor(...args: number[]) { |
| 64 | + this.args = args; |
| 65 | + } |
| 66 | + }; |
| 67 | + ``` |
| 68 | + |
| 69 | + ```ts |
| 70 | + // simplified |
| 71 | + const classGenerator = () => |
| 72 | + class DynamicGeneratedClass { |
| 73 | + args: number[]; |
| 74 | + constructor(...args: number[]) { |
| 75 | + this.args = args; |
| 76 | + } |
| 77 | + }; |
| 78 | + ``` |
| 79 | + |
| 80 | +4. avoid using `any` - there is no point at all to use typescript if you need to keep using `any`. |
| 81 | + |
| 82 | +This list will continue in future. |
| 83 | + |
| 84 | + </font> |
| 85 | + |
| 86 | +# Alternatives |
| 87 | + |
| 88 | +<font size="4"> |
| 89 | + |
| 90 | +1. You can use JS Doc @type for type definitions, typescript will work and check types for you |
| 91 | + |
| 92 | +2. You can always use JS + DTS - it is a similar way as it is done in C++ with .h and .cpp files |
| 93 | + DTS files are not working perfectly. Sometimes you forced to use JS Doc @typedef to import types from d.ts files |
| 94 | + |
| 95 | + </font> |
| 96 | + |
| 97 | +# Summary |
| 98 | + |
| 99 | +<font size="4"> |
| 100 | + |
| 101 | +I prefer to use JS + DTS or JS DOC + TypeScript, because it solve every type issues, but not requires to write code in TypeScript |
| 102 | + |
| 103 | +If it is not possible for you to follow this 2 solutions, please think about using those TS Guidelines. It will save you a lot of pain in future. |
| 104 | + |
| 105 | +TypeScript will sync their development withing the JavaScript standard. This means there will be no TS Decorators and TypeScript will become more like JS Extension rather than a different language transpiled to JS. |
| 106 | + |
| 107 | + </font> |
6 | 108 |
|
7 |
| - - **ecmascript** folder for ECMAScript Modules ts-configuration |
| 109 | +[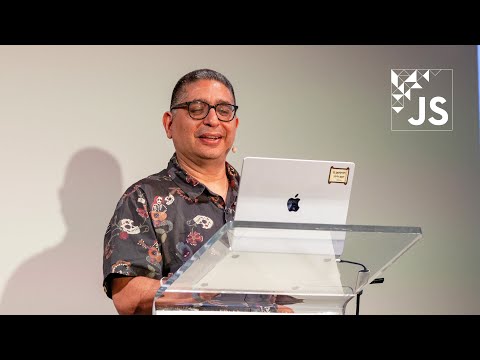](https://www.youtube.com/watch?v=SdV9Xy0E4CM) |
0 commit comments