-
Notifications
You must be signed in to change notification settings - Fork 40
Event Management Components
RC4Conferences is a powerful tool that allows you to easily create and manage virtual conferences. One of the key features of the platform is its modular design, which allows you to pick and choose the components that best suit your needs.
The event scheduler component is used to create and manage the schedule for your conference. It allows you to create sessions, assign speakers, and set the time and date for each session. You can also use it to create and manage tracks, which are groups of sessions that are related to a specific topic.
On any page, for example, pages/conferences/eventCreatePage.js
:
import Head from "next/head";
import { Stack } from "react-bootstrap";
import { useRouter } from "next/router";
import { EventCreate } from "../../../components/conferences/create/EventCreate";
import { fetchAPI } from "../../../lib/api";
function EventCreatePage() {
const router = useRouter();
const { eid } = router.query;
return (
<div>
<Head>
<title>Event Create</title>
<meta name="description" content="Event scheduler demo" />
<link rel="icon" href="/favicon.ico" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
</Head>
<div className="mx-auto">
<h1 className="mx-auto mt-3">Preview of Event Scheduler Component</h1>
<Stack direction="vertical">
<EventCreate active={eid} />
</Stack>
</div>
</div>
);
}
export async function getStaticPaths() {
return {
paths: [{ params: { eid: "basic-detail" }}, {params: {eid: "session"}}, {params: {eid: "other-details"}} ],
fallback: "blocking",
};
}
export async function getStaticProps(context) {
// load any custom data from your CMS
const topNavItems = await fetchAPI("/top-nav-item");
return {
props: { topNavItems },
revalidate: 10,
};
}
export default EventCreatePage;
Only users authenticated with the Open Event Server will be able to access this component since the event details are stored in Open Event Server's Backend. And to be able to read/write, we require the Auth token, which is stored in Cookies, and the component accesses it as follows:
let token = Cookies.get("event_auth");
token
? (token = JSON.parse(token).access_token)
: new Error("Please, Sign in again");
And the cookie should have the following structure:
{"access_token":"eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJpYXQiOjE2NzQ5MDMyMTcsIm5iZiI6MTY3NDkwMzIxNywianRpIjoiYzcwM2IwMjctNzJiZS00NjY1LThlODEtNDk3MDg4ZDQ1OWYyIiwiZXhwIjoxNjc0OTg5NjE3LCJpZGVudGl0eSI6MiwiZnJlc2giOnRydWUsInR5cGUiOiJhY2Nlc3MiLCJjc3JmIjoiMjNmYTA4MjQtN2NmMi00MmYwLTk5YTctMTRkNzE1Yjc2Y2RkIn0.1JrhLgUuZBaFKhOZmMzuW93iMcJFHsvXudKuMZWZUlk","jwtInfo":{"iat":1674903217,"nbf":1674903217,"jti":"c703b027-72be-4665-8e81-497088d459f2","exp":1674989617,"identity":2,"fresh":true,"type":"access","csrf":"23fa0824-7cf2-42f0-99a7-14d715b76cdd"}}
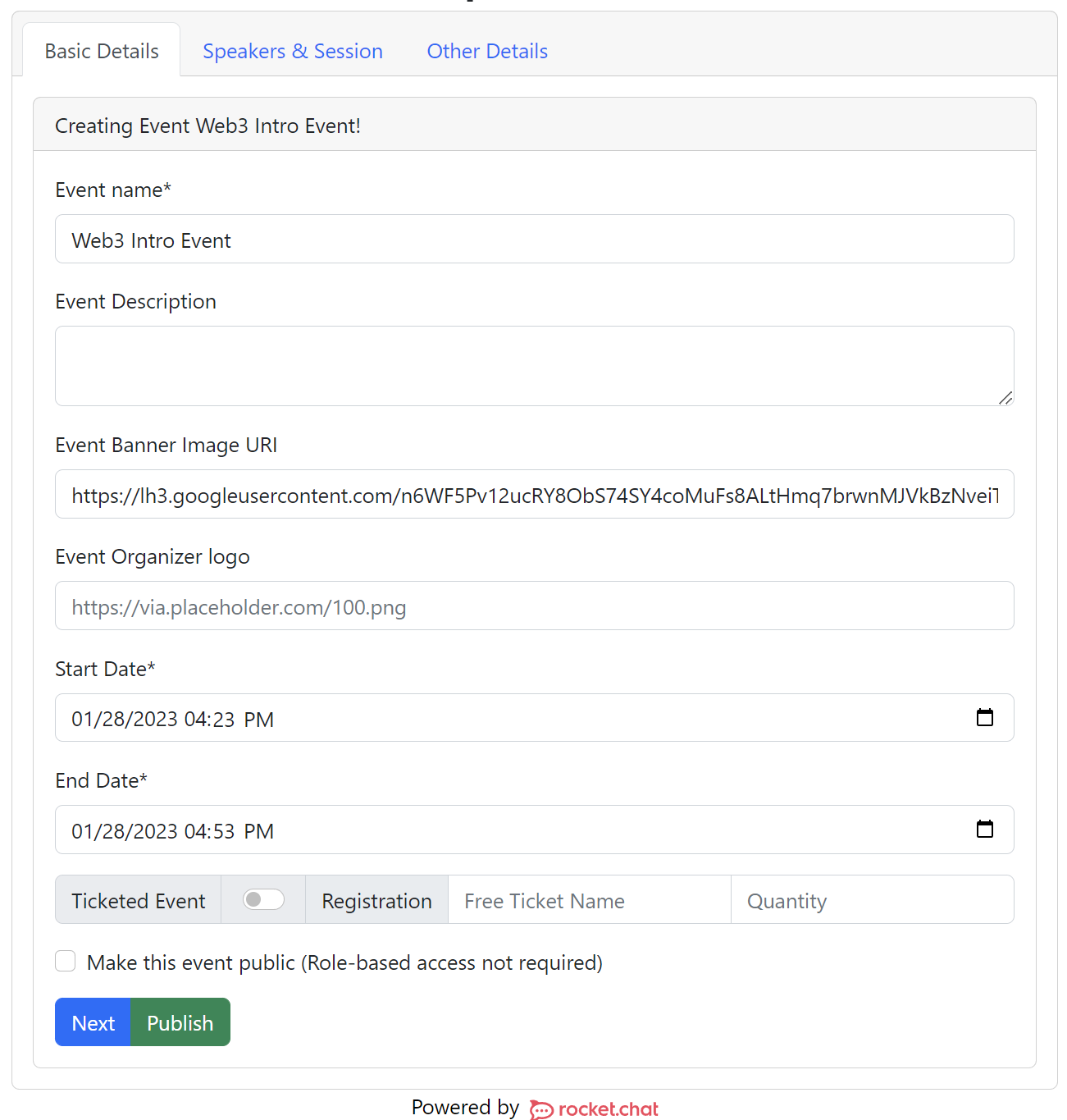